Sharing on iOS
Sharing on iOS is implemented by calling the methods SendMessage or Share with a FriendReqInfo
data structure, for Unity SDK or Unreal Engine SDK.
For more information on callbacks, see Callbacks.
Facebook
Since June 10, 2019, the Share to Messenger SDK is no longer available for new app integrations. However, existing projects can still use the SendMessage
method to share links to Messenger.
As new submissions are no longer accepted for the Web Games on Facebook and Facebook Gameroom platforms, Player Network SDK has discontinued support for sending invites with the SendMessage
method.
Facebook supports the following sharing modes:
- Sharing to Messenger: For sharing to Facebook Messenger.
- Sharing to Feed: For sharing to Facebook timeline.
- Sharing to Reels: For sharing to Facebook Reels.
- Sharing for Gaming: Specifically for gaming services, games are required to enable Login for Gaming to use this feature.
The following content can be shared to Facebook using SendMessage
and Share
:
- SendMessage: Link, for sharing to Messenger.
- Share: Link, for sharing to Feed.
- Share: Image, for sharing to Feed and sharing for Gaming.
- Share: Video, for sharing to Feed and sharing to Reels.
Since Facebook SDK V4.22.0, the Title
, Description
, Caption
and ImagePath
fields are no longer supported when sharing links.
SendMessage: Link
- Unity
- Unreal Engine
Call the SendMessage
method, with Type
set to INTLFriendReqType.Friend_REQ_LINK
.
The player must have installed the Messenger app on their device.
Parameters:
Type
Link
: URL
var reqInfo = new INTLFriendReqInfo();
reqInfo.Type = (int)INTLFriendReqType.Friend_REQ_LINK;
reqInfo.Link = "https://www.facebook.com/link";
INTLAPI.SendMessage(reqInfo, INTLChannel.Facebook);
Call the SendMessage
method, with Type
set to UINTLFriendReqType::kReqLink
.
The player must have installed the Messenger app on their device.
Parameters:
Type
Link
: URL
FINTLFriendReqInfo reqInfo;
reqInfo.Type = (int32)UINTLFriendReqType::kReqLink;
reqInfo.Link = "https://www.facebook.com/link";
UINTLSDKAPI::SendMessage(reqInfo, EINTLLoginChannel::kChannelFacebook);
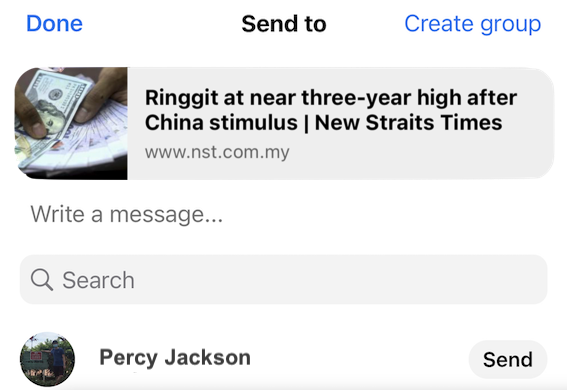
Share: Link
- Unity
- Unreal Engine
Call the Share
method, with Type
set to INTLFriendReqType.Friend_REQ_LINK
.
The player must have installed the Facebook app on their device.
Parameters:
Type
Link
: URLExtraJson
(Optional) -hashtag
- Each hashtag entered as "#value", only the first "#" will be highlighted.
var reqInfo = new INTLFriendReqInfo();
reqInfo.Type = (int)INTLFriendReqType.Friend_REQ_LINK;
reqInfo.Link = "https://www.facebook.com/link";
reqInfo.ExtraJson = "{\"hashtag \":\"#value\"}";
INTLAPI.Share(reqInfo, INTLChannel.Facebook);
Call the Share
method, with Type
set to UINTLFriendReqType::kReqLink
.
The player must have installed the Facebook app on their device.
Parameters:
Type
Link
: URLExtraJson
(Optional) -hashtag
- Each hashtag entered as "#value", only the first "#" will be highlighted.
FINTLFriendReqInfo reqInfo;
reqInfo.Type = (int32)UINTLFriendReqType::kReqLink;
reqInfo.Link = "https://www.facebook.com/link";
reqInfo.ExtraJson = "{\"hashtag \":\"#value\"}";
UINTLSDKAPI::Share(reqInfo, EINTLLoginChannel::kChannelFacebook);
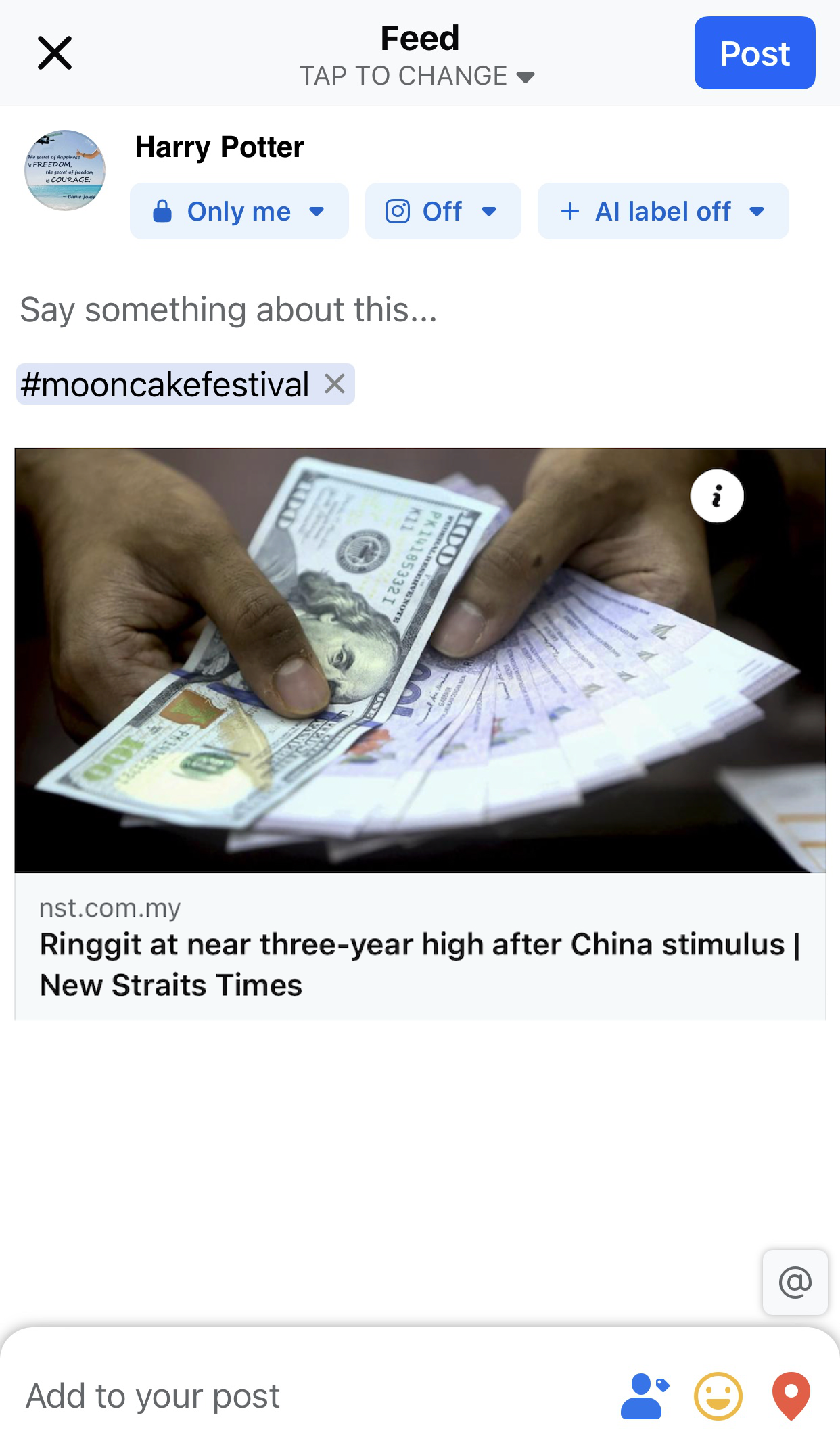
Share: Image
- Unity
- Unreal Engine
Call the Share
method, with Type
set to INTLFriendReqType.Friend_REQ_IMAGE
.
The player must have installed the Facebook app on their device.
Parameters:
Type
ImagePath
: Image file path, either the path of a local image file or an online URL.- Image cannot exceed 12MB.
- Online images will not be downloaded to local storage.
ExtraJson
(Optional) -hashtag
- Each hashtag entered as "#value", only the first "#" will be highlighted.
For sharing for Gaming, add the \"mode\":1
property to ExtraJson
. Hashtags cannot be added when sharing in this mode.
var reqInfo = new INTLFriendReqInfo();
reqInfo.Type = (int)INTLFriendReqType.Friend_REQ_IMAGE;
reqInfo.ImagePath = "https://www.google.com/images/branding/googlelogo/2x/googlelogo_color_272x92dp.png";
reqInfo.ExtraJson = "{\"hashtag \":\"#value\"}";
INTLAPI.Share(reqInfo, INTLChannel.Facebook);
Call the Share
method, with Type
set to UINTLFriendReqType::kReqImage
.
The player must have installed the Facebook app on their device.
Parameters:
Type
ImagePath
: Image file path, either the path of a local image file or an online URL.- Image cannot exceed 12MB.
- Online images will not be downloaded to local storage.
ExtraJson
(Optional) -hashtag
- Each hashtag entered as "#value", only the first "#" will be highlighted.
For sharing for Gaming, add the \"mode\":1
property to ExtraJson
. Hashtags cannot be added when sharing in this mode.
FINTLFriendReqInfo reqInfo ;
reqInfo.Type = (int32)UINTLFriendReqType::kReqImage,
reqInfo.ImagePath = "https://www.google.com/images/branding/googlelogo/2x/googlelogo_color_272x92dp.png";
reqInfo.ExtraJson = "{\"hashtag \":\"#value\"}";
UINTLSDKAPI::Share(reqInfo, EINTLLoginChannel::kChannelFacebook);
Sharing to Feed:
Sharing for Gaming:
Share: Video (Feed)
- Unity
- Unreal Engine
Call the Share
method, with Type
set to INTLFriendReqType.Friend_REQ_VIDEO
.
The player must have installed the Facebook app on their device.
Parameters:
Type
MediaPath
: Video file path, video cannot exceed 50MB.ExtraJson
(Optional) -hashtag
- Each hashtag entered as "#value", only the first "#" will be highlighted.
var reqInfo = new INTLFriendReqInfo();
reqInfo.Type = (int)INTLFriendReqType.Friend_REQ_VIDEO;
reqInfo.MediaPath = "/path/to/video";
reqInfo.ExtraJson = "{\"hashtag \":\"#value\"}";
INTLAPI.Share(reqInfo, INTLChannel.Facebook);
Call the Share
method, with Type
set to UINTLFriendReqType::kReqVideo
.
The player must have installed the Facebook app on their device.
Parameters:
Type
MediaPath
: Video file path, video cannot exceed 50MB.ExtraJson
(Optional) -hashtag
- Each hashtag entered as "#value", only the first "#" will be highlighted.
FINTLFriendReqInfo reqInfo ;
reqInfo.Type = (int32)UINTLFriendReqType::kReqVideo,
reqInfo.MediaPath = "/path/to/video";
reqInfo.ExtraJson = "{\"hashtag \":\"#value\"}";
UINTLSDKAPI::Share(reqInfo, EINTLLoginChannel::kChannelFacebook);
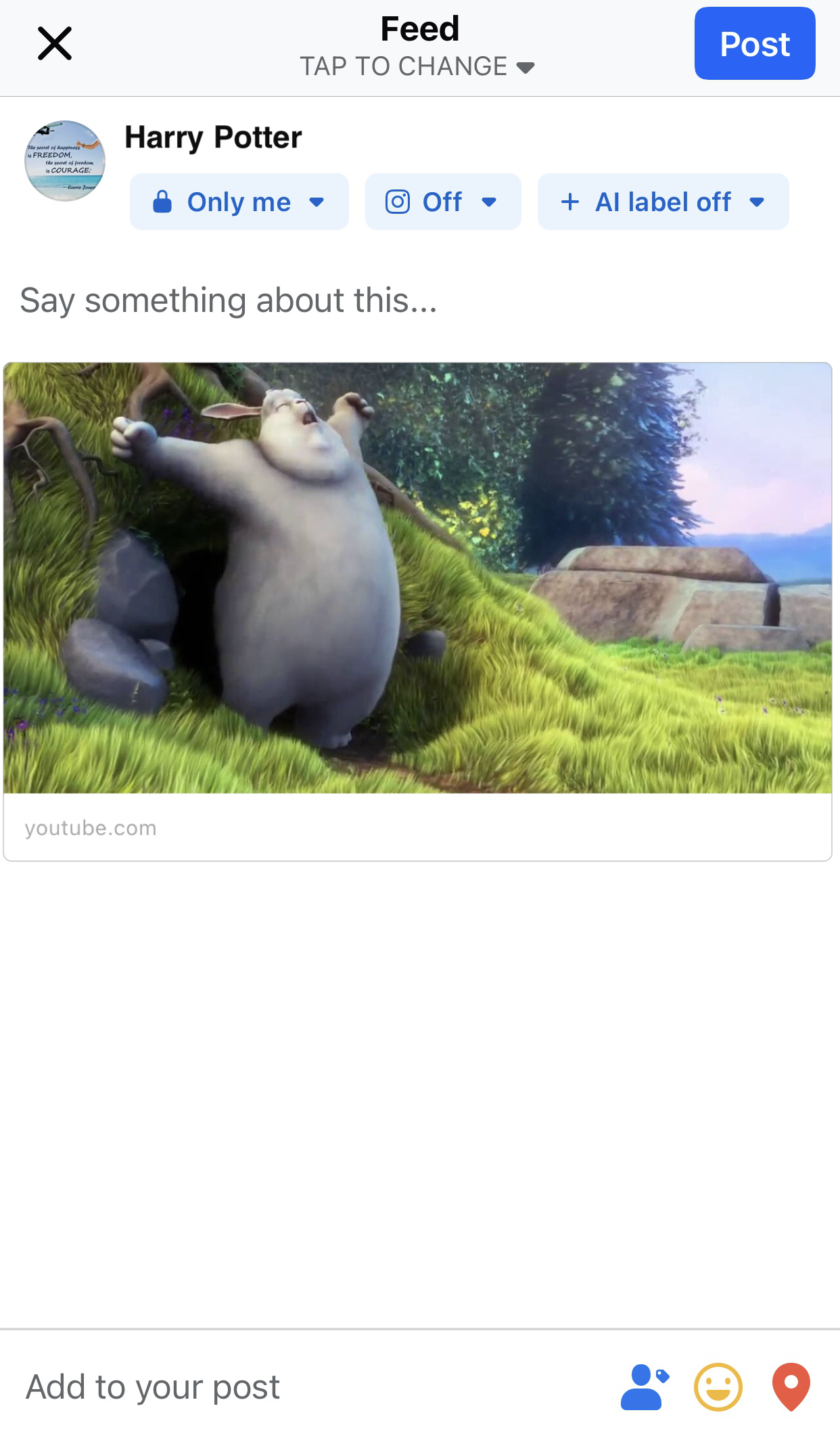
Share: Video (Reels)
- Unity
- Unreal Engine
Call the Share
method, with Type
set to INTLFriendReqType.Friend_REQ_VIDEO
.
The player must have installed the Facebook app on their device.
Parameters:
Type
MediaPath
: Video file path, video cannot exceed 50MB.ExtraJson
:share_to_reels
,hashtag
share_to_reels
: Set totrue
.hashtag
(Optional): Each hashtag entered as "#value", only the first "#" will be highlighted.
var reqInfo = new INTLFriendReqInfo();
reqInfo.Type = (int)INTLFriendReqType.Friend_REQ_VIDEO;
reqInfo.MediaPath = "/path/to/video";
reqInfo.ExtraJson = "{\"share_to_reels\":true, \"hashtag \":\"#value\"}";
INTLAPI.Share(reqInfo, INTLChannel.Facebook);
Call the Share
method, with Type
set to UINTLFriendReqType::kReqVideo
.
The player must have installed the Facebook app on their device.
Parameters:
Type
MediaPath
: Video file path, video cannot exceed 50MB.ExtraJson
:share_to_reels
,hashtag
share_to_reels
: Set totrue
.hashtag
(Optional): Each hashtag entered as "#value", only the first "#" will be highlighted.
FINTLFriendReqInfo reqInfo ;
reqInfo.Type = (int32)UINTLFriendReqType::kReqVideo,
reqInfo.MediaPath = "/path/to/video";
reqInfo.ExtraJson = "{\"share_to_reels\":true, \"hashtag \":\"#value\"}";
UINTLSDKAPI::Share(reqInfo, EINTLLoginChannel::kChannelFacebook);
Garena
When sharing player content to sub-channels supported by Garena, the channel name and required parameters can be passed to the properties of ExtraJson
in the FriendReqInfo
data structure when calling the SendMessage
or Share
methods. Supported sub-channels include Garena, Facebook, and LINE.
After sharing online images to a Garena sub-channel, the images will not be saved to the device local storage.
ExtraJson | Remarks |
---|---|
subChannel | Garena sub-channel name |
mediaTagName | String based on game requirement, may be empty |
caption | Description of the shared content |
Sub-channel Garena
The following content can be shared to sub-channel Garena using SendMessage
:
- SendMessage: Text
- SendMessage: Link
- SendMessage: Image
SendMessage: Text
- Unity
- Unreal Engine
Call the SendMessage
method, with Type
set to INTLFriendReqType.Friend_REQ_TEXT
.
The player must have installed the Garena app on their device and is currently logged in.
Parameters:
Type
Title
: TitleDescription
: Text contentThumbPath
: Thumbnail file pathExtraJson
-subChannel
,mediaTagName
var reqInfo = new INTLFriendReqInfo();
reqInfo.Type = (int)INTLFriendReqType.Friend_REQ_TEXT;
reqInfo.Title = "INTL share";
reqInfo.Description = "Hello, long time no see";
reqInfo.ThumbPath = "http://mat1.gtimg.com/www/qq2018/imgs/qq_logo_2018x2.png";
reqInfo.ExtraJson = "{\"subChannel\":\"Garena\",\"mediaTagName\":\"me\"}";
INTLAPI::SendMessage(friendInfo, "Garena");
While Garena does not support Player Network's invite type, invites can be sent via text messages, using mediaTagName
as the room ID.
When a friend clicks the invite, the game opens and the mediaTagName
value is passed through game_data
in ret.ExtraJson
during OnAuthBaseResultEvent
. The friend then joins the room.
Code sample:
public void OnAuthBaseResultEvent(INTLBaseResult baseRet)
{
if(baseRet.MethodId == (int)INTLMethodID.INTL_AUTH_WAKEUP)
{
// Listen to the team invitation callback
}
}
Call the SendMessage
method, with Type
set to UINTLFriendReqType::kReqText
.
The player must have installed the Garena app on their device and is currently logged in.
Parameters:
Type
Title
: TitleDescription
: Text contentThumbPath
: Thumbnail file pathExtraJson
-subChannel
,mediaTagName
FINTLFriendReqInfo reqInfo;
reqInfo.Type = (int32)UINTLFriendReqType::kReqText;
reqInfo.Title = "INTL share";
reqInfo.Description = "Hello, long time no see";
reqInfo.ThumbPath = "http://mat1.gtimg.com/www/qq2018/imgs/qq_logo_2018x2.png";
reqInfo.ExtraJson = "{\"subChannel\":\"Garena\",\"mediaTagName\":\"me\"}";
UINTLSDKAPI::SendMessage(reqInfo, "Garena");
While Garena does not support Player Network's invite type, invites can be sent via text messages, using mediaTagName
as the room ID.
When a friend clicks the invite, the game opens and the mediaTagName
value is passed through game_data
in ret.ExtraJson
during OnAuthBaseResultEvent
. The friend then joins the room.
Code sample:
// INTLAuthBaseResult callback
void INTLSDKAPIObserverImpl::OnAuthBaseResult_Implementation(FINTLBaseResult ret)
{
if(ret.MethodId == kMethodIDAuthWakeUp)
{
// Listen to the team invitation callback
}
}
Response sample:
{
"RetCode":0,
"RetMsg":"Success",
"MethodId":109,
"ThirdCode":-1,
"ThirdMsg":"",
"ExtraJson":"{\"params\":\"{\\\"platform\\\":\\\"1\\\",\\\"from_open_id\\\":\\\"f9aed405b81abfbdfeb305c431335ca3\\\",\\\"open_id\\\":\\\"9317b7044e2feaeff33d1feac2de33b4\\\"}\",\"game_data\":\"33\"}"
}
SendMessage: Link
- Unity
- Unreal Engine
Call the SendMessage
method, with Type
set to INTLFriendReqType.Friend_REQ_LINK
.
The player must have installed the Garena app on their device and is currently logged in.
Parameters:
Type
Link
: URLTitle
: TitleDescription
: DescriptionExtraJson
-subChannel
,mediaTagName
,caption
var reqInfo = new INTLFriendReqInfo();
reqInfo.Type = (int)INTLFriendReqType.Friend_REQ_LINK;
reqInfo.Link = "https://www.google.com";
reqInfo.Title = "INTL share";
reqInfo.Description = "Hello, long time no see";
reqInfo.ExtraJson = "{\"subChannel\":\"Garena\",\"mediaTagName\":\"me\",\"caption\":\"New Strait Times\"}";
INTLAPI::SendMessage(friendInfo, "Garena");
Call the SendMessage
method, with Type
set to UINTLFriendReqType::kReqLink
.
The player must have installed the Garena app on their device and is currently logged in.
Parameters:
Type
Link
: URLTitle
: TitleDescription
: DescriptionExtraJson
-subChannel
,mediaTagName
,caption
FINTLFriendReqInfo reqInfo;
reqInfo.Type = (int32)UINTLFriendReqType::kReqLink;
reqInfo.Link = "https://www.google.com";
reqInfo.Title = "INTL share";
reqInfo.Description = "Hello, long time no see";
reqInfo.ExtraJson = "{\"subChannel\":\"Garena\",\"mediaTagName\":\"me\",\"caption\":\"New Strait Times\"}";
UINTLSDKAPI::SendMessage(reqInfo, "Garena");
SendMessage: Image
- Unity
- Unreal Engine
Call the SendMessage
method, with Type
set to INTLFriendReqType.Friend_REQ_IMAGE
.
The player must have installed the Garena app on their device and is currently logged in.
Parameters:
Type
ImagePath
: Image file pathExtraJson
-subChannel
,mediaTagName
var reqInfo = new INTLFriendReqInfo();
reqInfo.Type = (int)INTLFriendReqType.Friend_REQ_IMAGE;
reqInfo.ImagePath = "https://www.google.com/images/branding/googlelogo/2x/googlelogo_color_272x92dp.png";
reqInfo.ExtraJson = "{\"subChannel\":\"Garena\",\"mediaTagName\":\"me\"}";
INTLAPI::SendMessage(friendInfo, "Garena");
Call the SendMessage
method, with Type
set to UINTLFriendReqType::kReqImage
.
The player must have installed the Garena app on their device and is currently logged in.
Parameters:
Type
ImagePath
: Image file pathExtraJson
-subChannel
,mediaTagName
FINTLFriendReqInfo reqInfo;
reqInfo.Type = (int32)UINTLFriendReqType::kReqImage;
reqInfo.ImagePath = "https://www.google.com/images/branding/googlelogo/2x/googlelogo_color_272x92dp.png";
reqInfo.ExtraJson = "{\"subChannel\":\"Garena\",\"mediaTagName\":\"me\"}";
UINTLSDKAPI::SendMessage(reqInfo, "Garena");
Sub-channel Facebook
The following content can be shared to sub-channel Facebook using Share
:
- Share: Text
- Share: Link
- Share: Image
Since Facebook SDK V4.22.0, the Title
, Description
, Caption
and ImagePath
fields are no longer supported when sharing links.
Share: Text
- Unity
- Unreal Engine
Call the Share
method, with Type
set to INTLFriendReqType.Friend_REQ_TEXT
.
The player must have installed the Facebook app on their device.
Parameters:
Type
Description
: Text contentExtraJson
-subChannel
var reqInfo = new INTLFriendReqInfo();
reqInfo.Type = (int)INTLFriendReqType.Friend_REQ_TEXT;
reqInfo.Description = "Hello, long time no see";
reqInfo.ExtraJson = "{\"subChannel\":\"Facebook\"}";
INTLAPI::Share(friendInfo, "Garena");
Call the Share
method, with Type
set to UINTLFriendReqType::kReqText
.
The player must have installed the Facebook app on their device.
Parameters:
Type
Description
: Text contentExtraJson
-subChannel
FINTLFriendReqInfo reqInfo;
reqInfo.Type = (int32)UINTLFriendReqType::kReqText;
reqInfo.Description = "Hello, long time no see";
reqInfo.ExtraJson = "{\"subChannel\":\"Facebook\"}";
UINTLSDKAPI::Share(reqInfo, "Garena");
Share: Link
- Unity
- Unreal Engine
Call the Share
method, with Type
set to INTLFriendReqType.Friend_REQ_LINK
.
The player must have installed the Facebook app on their device.
Parameters:
Type
Link
: URLExtraJson
-subChannel
var reqInfo = new INTLFriendReqInfo();
reqInfo.Type = (int)INTLFriendReqType.Friend_REQ_LINK;
reqInfo.Link = "https://www.google.com";
reqInfo.ExtraJson = "{\"subChannel\":\"Facebook\"}";
INTLAPI::Share(friendInfo, "Garena");
Call the Share
method, with Type
set to UINTLFriendReqType::kReqLink
.
The player must have installed the Facebook app on their device.
Parameters:
Type
Link
: URLExtraJson
-subChannel
FINTLFriendReqInfo reqInfo;
reqInfo.Type = (int32)UINTLFriendReqType::kReqLink;
reqInfo.Link = "https://www.google.com";
reqInfo.ExtraJson = "{\"subChannel\":\"Facebook\"}";
UINTLSDKAPI::Share(reqInfo, "Garena");
Share: Image
- Unity
- Unreal Engine
Call the Share
method, with Type
set to INTLFriendReqType.Friend_REQ_IMAGE
.
The player must have installed the Facebook app on their device.
Parameters:
Type
ImagePath
: Image file path, image cannot exceed 12MBExtraJson
-subChannel
,mediaTagName
var reqInfo = new INTLFriendReqInfo();
reqInfo.Type = (int)INTLFriendReqType.Friend_REQ_IMAGE;
reqInfo.ImagePath = "https://www.google.com/images/branding/googlelogo/2x/googlelogo_color_272x92dp.png";
reqInfo.ExtraJson = "{\"subChannel\":\"Facebook\",\"mediaTagName\":\"me\"}";
INTLAPI::Share(friendInfo, "Garena");
Call the Share
method, with Type
set to UINTLFriendReqType::kReqImage
.
The player must have installed the Facebook app on their device.
Parameters:
Type
ImagePath
: Image file path, image cannot exceed 12MBExtraJson
-subChannel
,mediaTagName
FINTLFriendReqInfo reqInfo;
reqInfo.Type = (int32)UINTLFriendReqType::kReqImage;
reqInfo.ImagePath = "https://www.google.com/images/branding/googlelogo/2x/googlelogo_color_272x92dp.png";
reqInfo.ExtraJson = "{\"subChannel\":\"Facebook\",\"mediaTagName\":\"me\"}";
UINTLSDKAPI::Share(reqInfo, "Garena");
Sub-channel LINE
The following content can be shared to sub-channel LINE using SendMessage
and Share
:
- SendMessage: Link
- SendMessage: Image
- Share: Link
- Share: Image
SendMessage: Link
- Unity
- Unreal Engine
Call the SendMessage
method, with Type
set to INTLFriendReqType.Friend_REQ_LINK
.
The player must have installed the LINE app on their device, but does not require Garena to be logged in.
Parameters:
Type
Link
: URLExtraJson
-subChannel
var reqInfo = new INTLFriendReqInfo();
reqInfo.Type = (int)INTLFriendReqType.Friend_REQ_LINK;
reqInfo.Link = "https://www.google.com";
reqInfo.ExtraJson = "{\"subChannel\":\"Line\"}";
INTLAPI::SendMessage(friendInfo, "Garena");
Call the SendMessage
method, with Type
set to UINTLFriendReqType::kReqLink
.
The player must have installed the LINE app on their device, but does not require Garena to be logged in.
Parameters:
Type
Link
: URLExtraJson
-subChannel
FINTLFriendReqInfo reqInfo;
reqInfo.Type = (int32)UINTLFriendReqType::kReqLink;
reqInfo.Link = "https://www.google.com";
reqInfo.ExtraJson = "{\"subChannel\":\"Line\"}";
UINTLSDKAPI::SendMessage(reqInfo, "Garena");
SendMessage: Image
- Unity
- Unreal Engine
Call the SendMessage
method, with Type
set to INTLFriendReqType.Friend_REQ_IMAGE
.
The player must have installed the LINE app on their device, but does not require Garena to be logged in.
Parameters:
Type
ImagePath
: Image file pathExtraJson
-subChannel
var reqInfo = new INTLFriendReqInfo();
reqInfo.Type = (int)INTLFriendReqType.Friend_REQ_IMAGE;
reqInfo.ImagePath = "https://www.google.com/images/branding/googlelogo/2x/googlelogo_color_272x92dp.png";
reqInfo.ExtraJson = "{\"subChannel\":\"Line\"}";
INTLAPI::SendMessage(friendInfo, "Garena");
Call the SendMessage
method, with Type
set to UINTLFriendReqType::kReqImage
.
The player must have installed the LINE app on their device, but does not require Garena to be logged in.
Parameters:
Type
ImagePath
: Image file pathExtraJson
-subChannel
FINTLFriendReqInfo reqInfo;
reqInfo.Type = (int32)UINTLFriendReqType::kReqImage;
reqInfo.ImagePath = "https://www.google.com/images/branding/googlelogo/2x/googlelogo_color_272x92dp.png";
reqInfo.ExtraJson = "{\"subChannel\":\"Line\"}";
UINTLSDKAPI::SendMessage(reqInfo, "Garena");
Share: Link
- Unity
- Unreal Engine
Call the Share
method, with Type
set to INTLFriendReqType.Friend_REQ_LINK
.
The player must have installed the LINE app on their device, but does not require Garena to be logged in.
Parameters:
Type
Link
: URLExtraJson
-subChannel
var reqInfo = new INTLFriendReqInfo();
reqInfo.Type = (int)INTLFriendReqType.Friend_REQ_LINK;
reqInfo.Link = "https://www.google.com";
reqInfo.ExtraJson = "{\"subChannel\":\"Line\"}";
INTLAPI::Share(friendInfo, "Garena");
Call the Share
method, with Type
set to UINTLFriendReqType::kReqLink
.
The player must have installed the LINE app on their device, but does not require Garena to be logged in.
Parameters:
Type
Link
: URLExtraJson
-subChannel
FINTLFriendReqInfo reqInfo;
reqInfo.Type = (int32)UINTLFriendReqType::kReqLink;
reqInfo.Link = "https://www.google.com";
reqInfo.ExtraJson = "{\"subChannel\":\"Line\"}";
UINTLSDKAPI::Share(reqInfo, "Garena");
Share: Image
- Unity
- Unreal Engine
Call the Share
method, with Type
set to INTLFriendReqType.Friend_REQ_IMAGE
.
The player must have installed the LINE app on their device, but does not require Garena to be logged in.
Parameters:
Type
ImagePath
: Image file pathExtraJson
-subChannel
var reqInfo = new INTLFriendReqInfo();
reqInfo.Type = (int)INTLFriendReqType.Friend_REQ_IMAGE;
reqInfo.ImagePath = "https://www.google.com/images/branding/googlelogo/2x/googlelogo_color_272x92dp.png";
reqInfo.ExtraJson = "{\"subChannel\":\"Line\"}";
INTLAPI::Share(friendInfo, "Garena");
Call the Share
method, with Type
set to UINTLFriendReqType::kReqImage
.
The player must have installed the LINE app on their device, but does not require Garena to be logged in.
Parameters:
Type
ImagePath
: Image file pathExtraJson
-subChannel
FINTLFriendReqInfo reqInfo;
reqInfo.Type = (int32)UINTLFriendReqType::kReqImage;
reqInfo.ImagePath = "https://www.google.com/images/branding/googlelogo/2x/googlelogo_color_272x92dp.png";
reqInfo.ExtraJson = "{\"subChannel\":\"Line\"}";
UINTLSDKAPI::Share(reqInfo, "Garena");
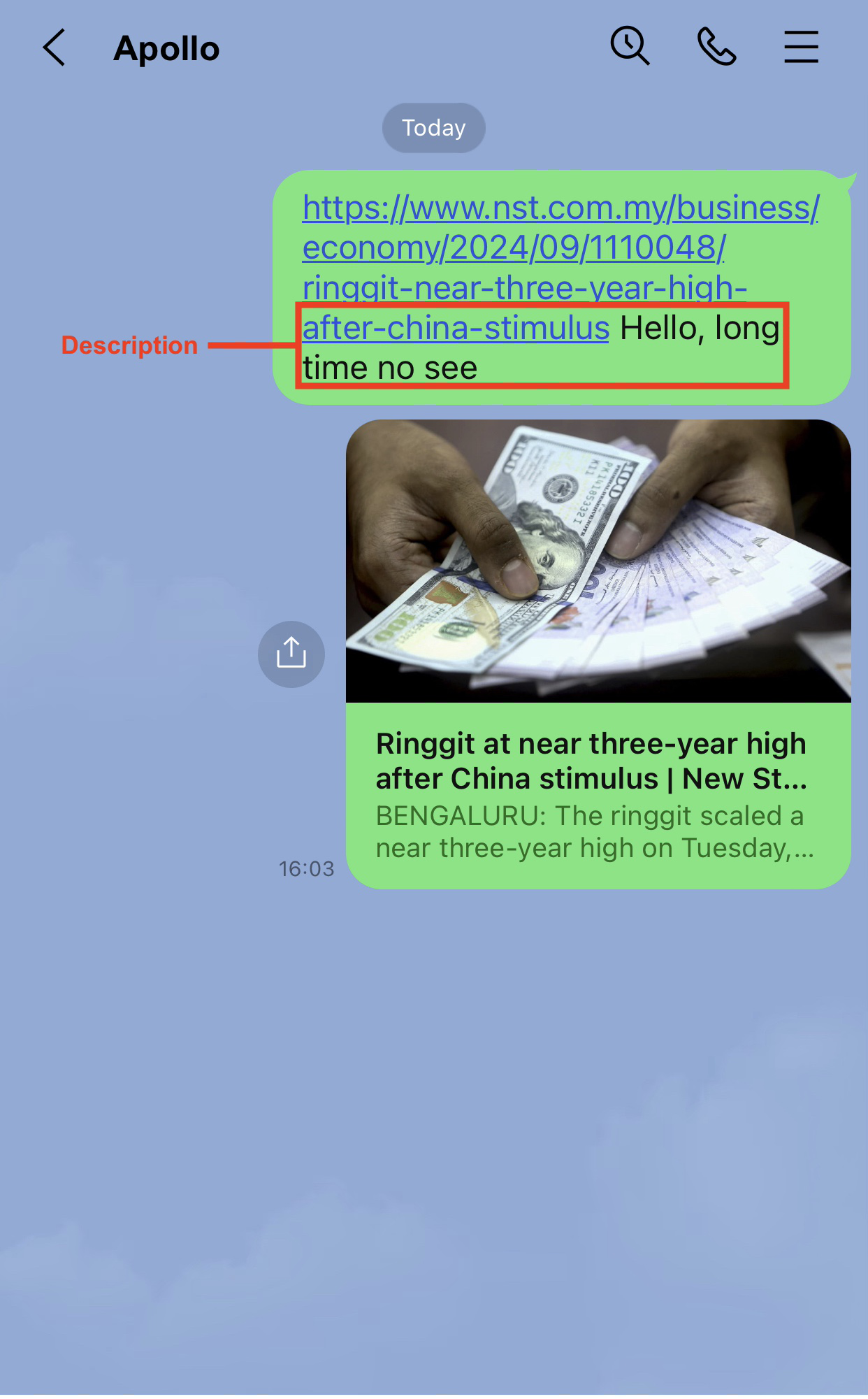
Instagram
The following content can be shared to feed, stories, and messages on Instagram using Share
:
- Share: Image
- Share: Video
The Instagram sharing feature requires iOS 15.1 or later. For more information, see the Instagram app on the App Store.
Share: Image
- Unity
- Unreal Engine
Call the Share
method, with Type
set to INTLFriendReqType.Friend_REQ_IMAGE
.
The player must have installed the Instagram app on their device.
Parameters:
Type
ImagePath
: Image file path, either the path of a local image file or an online URL.- Preferred format: JPEG (640 x 640 pixels)
var reqInfo = new INTLFriendReqInfo();
reqInfo.Type = (int)INTLFriendReqType.Friend_REQ_IMAGE;
reqInfo.ImagePath = "/path/to/image";
INTLAPI.Share(reqInfo, INTLChannel.Instagram);
Call the Share
method, with Type
set to UINTLFriendReqType::kReqImage
.
The player must have installed the Instagram app on their device.
Parameters:
Type
ImagePath
: Image file path, either the path of a local image file or an online URL.- Preferred format: JPEG (640 x 640 pixels)
FINTLFriendReqInfo reqInfo ;
reqInfo.Type = (int32)UINTLFriendReqType::kReqImage;
reqInfo.ImagePath = "/path/to/image";
UINTLSDKAPI::Share(reqInfo, EINTLLoginChannel::kChannelInstagram);
Share: Video
- Unity
- Unreal Engine
Call the Share
method, with Type
set to INTLFriendReqType.Friend_REQ_VIDEO
.
The player must have installed the Instagram app on their device.
Parameters:
Type
MediaPath
: Video file path, either the path of a local video file or an online URL.- Format: MP4
- Min duration: 3s, Max duration: 10mins
- Min dimensions: 640 x 640 pixels
var reqInfo = new INTLFriendReqInfo();
reqInfo.Type = (int)INTLFriendReqType.Friend_REQ_VIDEO;
reqInfo.MediaPath = "/path/to/video";
INTLAPI.Share(reqInfo, INTLChannel.Instagram);
Call the Share
method, with Type
set to UINTLFriendReqType::kReqVideo
.
The player must have installed the Instagram app on their device.
Parameters:
Type
MediaPath
: Video file path, either the path of a local video file or an online URL.- Format: MP4
- Min duration: 3s, Max duration: 10mins
- Min dimensions: 640 x 640 pixels
FINTLFriendReqInfo reqInfo ;
reqInfo.Type = (int32)UINTLFriendReqType::kReqVideo;
reqInfo.MediaPath = "/path/to/video";
UINTLSDKAPI::Share(reqInfo, EINTLLoginChannel::kChannelInstagram);
LINE
The following content can be shared to LINE using SendMessage
:
- SendMessage: Text
- SendMessage: Image
SendMessage: Text
- Unity
- Unreal Engine
Call the SendMessage
method, with Type
set to INTLFriendReqType.Friend_REQ_TEXT
.
Parameters:
Type
Description
: Text content
var reqInfo = new INTLFriendReqInfo();
reqInfo.Type = (int)INTLFriendReqType.Friend_REQ_TEXT;
reqInfo.Description = "INTL services";
INTLAPI.SendMessage(reqInfo, INTLChannel.Line);
Call the SendMessage
method, with Type
set to UINTLFriendReqType::kReqText
.
Parameters:
Type
Description
: Text content
FINTLFriendReqInfo reqInfo;
reqInfo.Type = (int32)UINTLFriendReqType::kReqText;
reqInfo.Description = "INTL services";
UINTLSDKAPI::SendMessage(reqInfo, UINTLLoginChannel::kChannelLine);
SendMessage: Image
- Unity
- Unreal Engine
Call the SendMessage
method, with Type
set to INTLFriendReqType.Friend_REQ_IMAGE
.
Parameters:
Type
ImagePath
: Image file path, either the path of a local image file or an online URL.
var reqInfo = new INTLFriendReqInfo();
reqInfo.Type = (int)INTLFriendReqType.Friend_REQ_IMAGE;
reqInfo.ImagePath = "https://www.google.com/images/branding/googlelogo/2x/googlelogo_color_272x92dp.png";
INTLAPI.SendMessage(reqInfo, INTLChannel.Line);
Call the SendMessage
method, with Type
set to UINTLFriendReqType::kReqImage
.
Parameters:
Type
ImagePath
: Image file path, either the path of a local image file or an online URL.
FINTLFriendReqInfo reqInfo ;
reqInfo.Type = (int32)UINTLFriendReqType::kReqImage;
reqInfo.ImagePath = "https://www.google.com/images/branding/googlelogo/2x/googlelogo_color_272x92dp.png";
UINTLSDKAPI::SendMessage(reqInfo, UINTLLoginChannel::kChannelLine);
Error code
- Unity
- Unreal Engine
void CheckThirdCode(INTLAuthResult result)
{
if(result.RetCode == 9999)
{
switch(result.ThirdCode):
{
case 5:
//LineSDKInternalErrorCodeInvalidTokenType
break;
case 2:
//LineSDKInternalErrorCodeMissingConfiguration
break;
case 2001:
// Error happens in the underlying `URLSession`.
break;
default:
// Unknown error code
break;
}
}
}
void CheckThirdCode(FINTLAuthResult result)
{
if(result.RetCode == 9999)
{
switch(result.ThirdCode):
{
case 5:
//LineSDKInternalErrorCodeInvalidTokenType
break;
case 2:
//LineSDKInternalErrorCodeMissingConfiguration
break;
case 2001:
// Error happens in the underlying `URLSession`.
break;
default:
// Unknown error code
break;
}
}
}
Error code | Error information |
---|---|
0 | Unknown error |
1 | Login error |
2 | Missing configuration |
3 | Verification canceled |
4 | Token loss |
5 | Incorrect token type |
6 | Missing required field |
7 | Invalid value type |
8 | Invalid web key type in JSON |
9 | Token ID verification failed |
For more information, see Handling errors.
QQ
QQ supports the following sharing modes:
- Sharing to QQ Friends: For sharing to QQ friends and groups using the
SendMessage
method. - Sharing to Qzone: For sharing to Qzone using the
Share
method.
The following content can be shared to QQ using SendMessage
and Share
:
- SendMessage: Text
- SendMessage: Link
- SendMessage: Image
- SendMessage: Video
- Share: Link
- Share: Image
- Share: Video
SendMessage: Text
- Unity
- Unreal Engine
Call the SendMessage
method, with Type
set to INTLFriendReqType.Friend_REQ_TEXT
.
The player must have installed the QQ app on their device and is currently logged in.
Parameters:
Type
Description
: Text content, max 1,536 characters
var reqInfo = new INTLFriendReqInfo();
reqInfo.Type = (int)INTLFriendReqType.Friend_REQ_TEXT;
reqInfo.Description = "INTL text sending test";
INTLAPI.SendMessage(reqInfo, INTLChannel.QQ);
Call the SendMessage
method, with Type
set to UINTLFriendReqType::kReqText
.
The player must have installed the QQ app on their device and is currently logged in.
Parameters:
Type
Description
: Text content, max 1,536 characters
FINTLFriendReqInfo reqInfo;
reqInfo.Type = (int32)UINTLFriendReqType::kReqText;
reqInfo.Description = "INTL text sending test";
UINTLSDKAPI::SendMessage(reqInfo, EINTLLoginChannel::kChannelQQ);
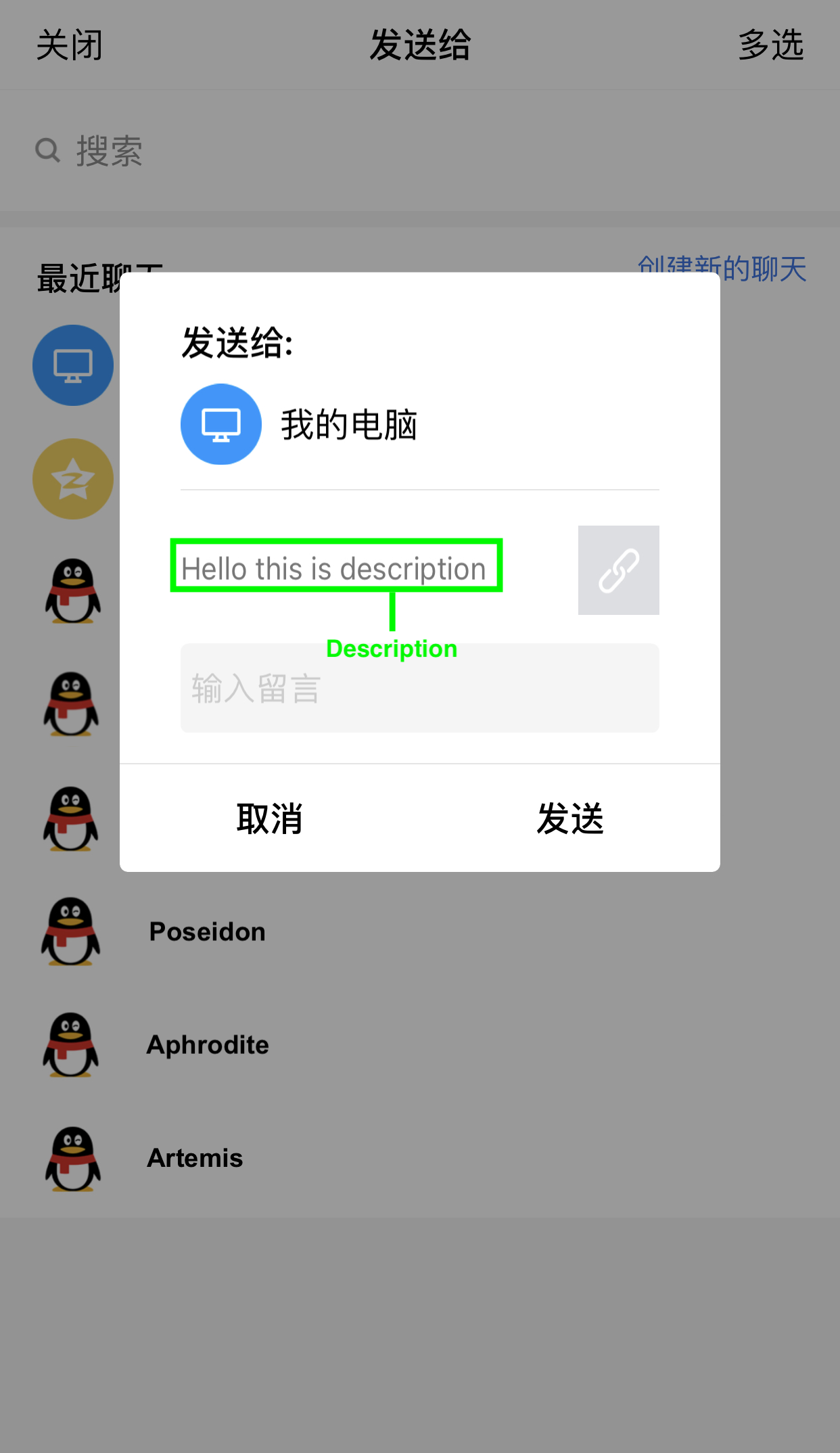
SendMessage: Link
- Unity
- Unreal Engine
Call the SendMessage
method, with Type
set to INTLFriendReqType.Friend_REQ_LINK
.
The player must have installed the QQ app on their device and is currently logged in.
Parameters:
Type
Title
: Title, max 128 charactersDescription
: Description, max 512 charactersLink
: URL, max 1,024 charactersThumbPath
: Thumbnail file path, image cannot exceed 1MB
var reqInfo = new INTLFriendReqInfo();
reqInfo.Type = (int)INTLFriendReqType.Friend_REQ_LINK;
reqInfo.Link = "https://www.google.com";
reqInfo.Title = "INTL test";
reqInfo.Description = "INTL link sending test";
reqInfo.ThumbPath = "http://mat1.gtimg.com/www/qq2018/imgs/qq_logo_2018x2.png";
INTLAPI.SendMessage(reqInfo, INTLChannel.QQ);
Call the SendMessage
method, with Type
set to UINTLFriendReqType::kReqLink
.
The player must have installed the QQ app on their device and is currently logged in.
Parameters:
Type
Title
: Title, max 128 charactersDescription
: Description, max 512 charactersLink
: URL, max 1,024 charactersThumbPath
: Thumbnail file path, image cannot exceed 1MB
FINTLFriendReqInfo reqInfo;
reqInfo.Type = (int32)UINTLFriendReqType::kReqLink;
reqInfo.Link = "https://www.google.com";
reqInfo.Title = "INTL test";
reqInfo.Description = "INTL link sending test";
reqInfo.ThumbPath = "http://mat1.gtimg.com/www/qq2018/imgs/qq_logo_2018x2.png";
UINTLSDKAPI::SendMessage(reqInfo, EINTLLoginChannel::kChannelQQ);
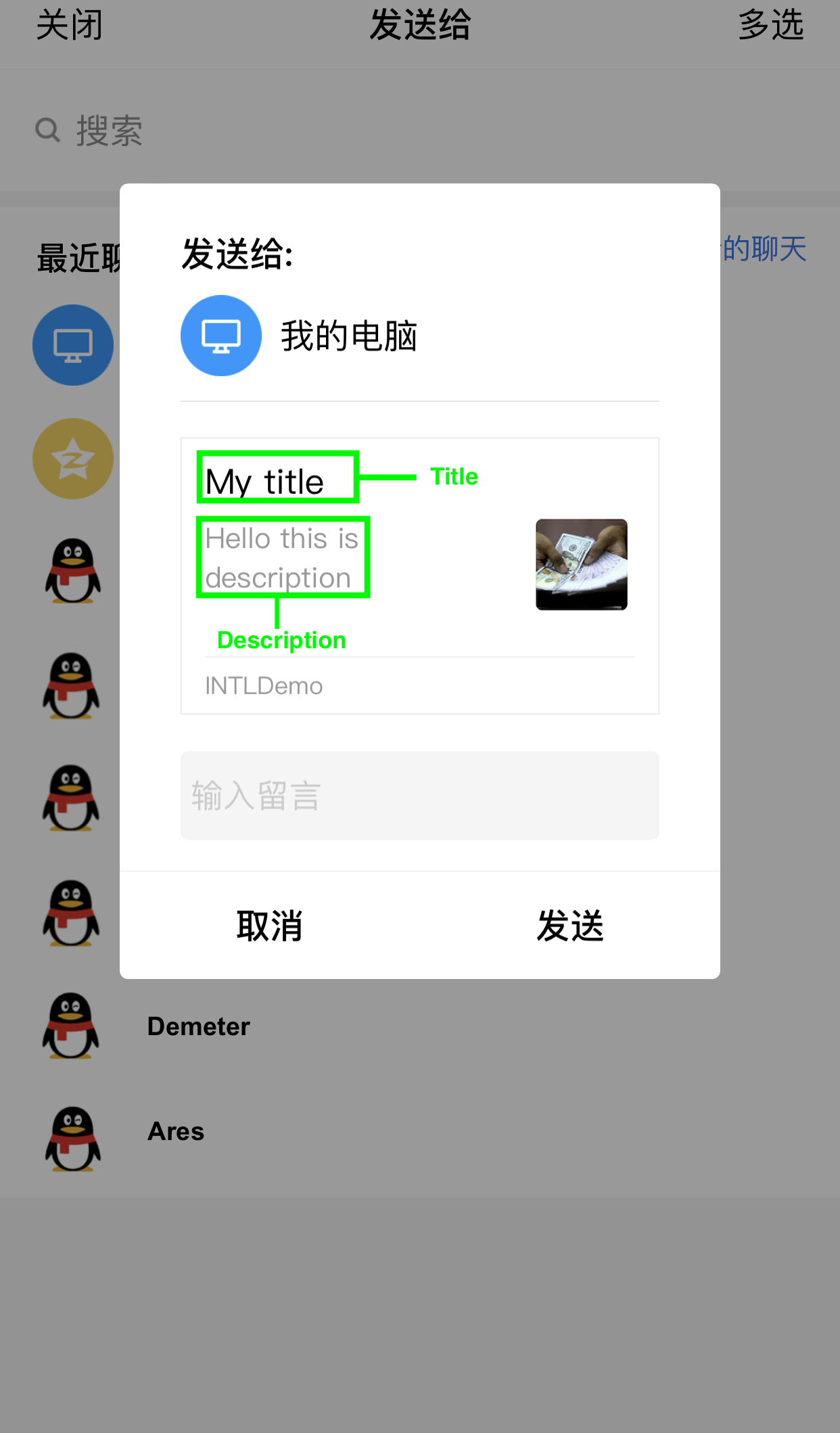
SendMessage: Image
- Unity
- Unreal Engine
Call the SendMessage
method, with Type
set to INTLFriendReqType.Friend_REQ_IMAGE
.
The player must have installed the QQ app on their device and is currently logged in.
Parameters:
Type
Title
: TitleDescription
: DescriptionImagePath
: Image file path, either the path of a local image file or an online URL
var reqInfo = new INTLFriendReqInfo();
reqInfo.Type = (int)INTLFriendReqType.Friend_REQ_IMAGE;
reqInfo.Title = "INTL test";
reqInfo.Description = "INTL description";
reqInfo.ImagePath = "http://mat1.gtimg.com/www/qq2018/imgs/qq_logo_2018x2.png";
INTLAPI.SendMessage(reqInfo, INTLChannel.QQ);
Call the SendMessage
method, with Type
set to UINTLFriendReqType::kReqImage
.
The player must have installed the QQ app on their device and is currently logged in.
Parameters:
Type
Title
: TitleDescription
: DescriptionImagePath
: Image file path, either the path of a local image file or an online URL
FINTLFriendReqInfo reqInfo ;
reqInfo.Type = (int32)UINTLFriendReqType::kReqImage;
reqInfo.Title = "INTL test";
reqInfo.Description = "INTL description";
reqInfo.ImagePath = "http://mat1.gtimg.com/www/qq2018/imgs/qq_logo_2018x2.png";
UINTLSDKAPI::SendMessage(reqInfo, EINTLLoginChannel::kChannelQQ);
SendMessage: Video
- Unity
- Unreal Engine
Call the SendMessage
method, with Type
set to INTLFriendReqType.Friend_REQ_VIDEO
.
The player must have installed the QQ app on their device and is currently logged in.
Parameters:
Type
Title
: TitleDescription
: DescriptionMediaPath
: Video file path, needs to be the URL of an online video
var reqInfo = new INTLFriendReqInfo ();
reqInfo.Type = (int)INTLFriendReqType.Friend_REQ_VIDEO;
reqInfo.Title = "Game Video";
reqInfo.Description = "Golden moment";
reqInfo.MediaPath ="http://v.qq.com/cover/5/53x6bbyb07ebl3s/n0013r8esy6.html";
INTLAPI.SendMessage(reqInfo, INTLChannel.QQ);
Call the SendMessage
method, with Type
set to UINTLFriendReqType::kReqVideo
.
The player must have installed the QQ app on their device and is currently logged in.
Parameters:
Type
Title
: TitleDescription
: DescriptionMediaPath
: Video file path, needs to be the URL of an online video
FINTLFriendReqInfo reqInfo ;
reqInfo.Type = (int32)UINTLFriendReqType::kReqVideo;
reqInfo.Title = "Game Video";
reqInfo.Description = "Golden moment";
reqInfo.MediaPath = "http://v.qq.com/cover/5/53x6bbyb07ebl3s/n0013r8esy6.html";
UINTLSDKAPI::SendMessage(reqInfo, EINTLLoginChannel::kChannelQQ);
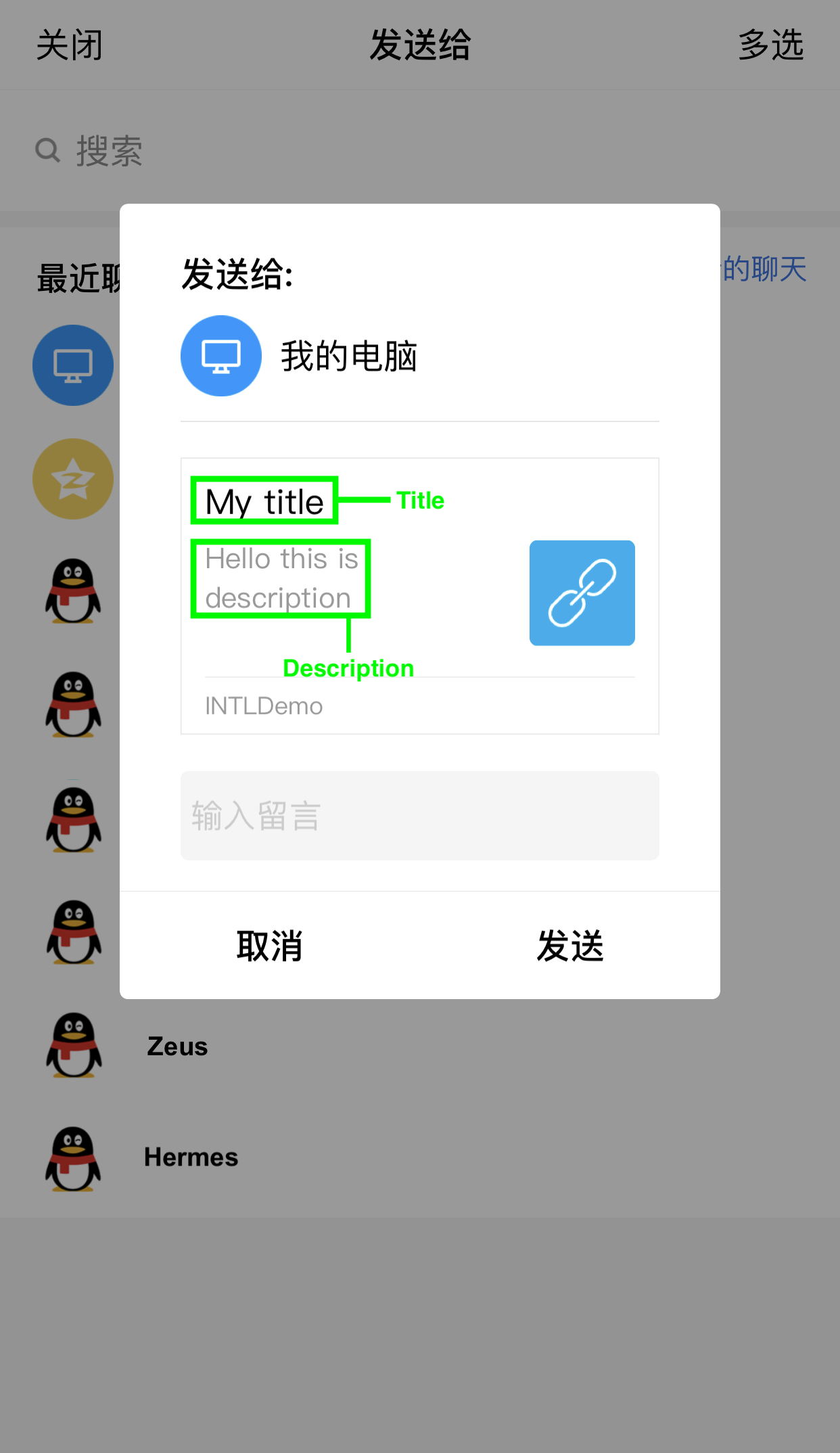
Share: Link
- Unity
- Unreal Engine
Call the Share
method, with Type
set to INTLFriendReqType.Friend_REQ_LINK
.
The player must have installed the QQ app on their device and is currently logged in.
Parameters:
Type
Title
: Title, max 128 charactersDescription
: Description, max 512 charactersLink
: URL, max 1,024 charactersImagePath
: Thumbnail file path, image cannot exceed 1MB
var reqInfo = new INTLFriendReqInfo ();
reqInfo.Type = (int)INTLFriendReqType.Friend_REQ_LINK;
reqInfo.Title = "INTL share";
reqInfo.Description = "INTL description";
reqInfo.Link = "https://www.google.com";
reqInfo.ImagePath = "http://mat1.gtimg.com/www/qq2018/imgs/qq_logo_2018x2.png";
INTLAPI.Share(reqInfo, INTLChannel.QQ);
Call the Share
method, with Type
set to UINTLFriendReqType::kReqLink
.
The player must have installed the QQ app on their device and is currently logged in.
Parameters:
Type
Title
: Title, max 128 charactersDescription
: Description, max 512 charactersLink
: URL, max 1,024 charactersImagePath
: Thumbnail file path, image cannot exceed 1MB
FINTLFriendReqInfo reqInfo;
reqInfo.Type = (int32)UINTLFriendReqType::kReqLink;
reqInfo.Title = "INTL share";
reqInfo.Description = "INTL description";
reqInfo.Link = "https://www.google.com";
reqInfo.ImagePath = "http://mat1.gtimg.com/www/qq2018/imgs/qq_logo_2018x2.png";
UINTLSDKAPI::Share(reqInfo, EINTLLoginChannel::kChannelQQ);
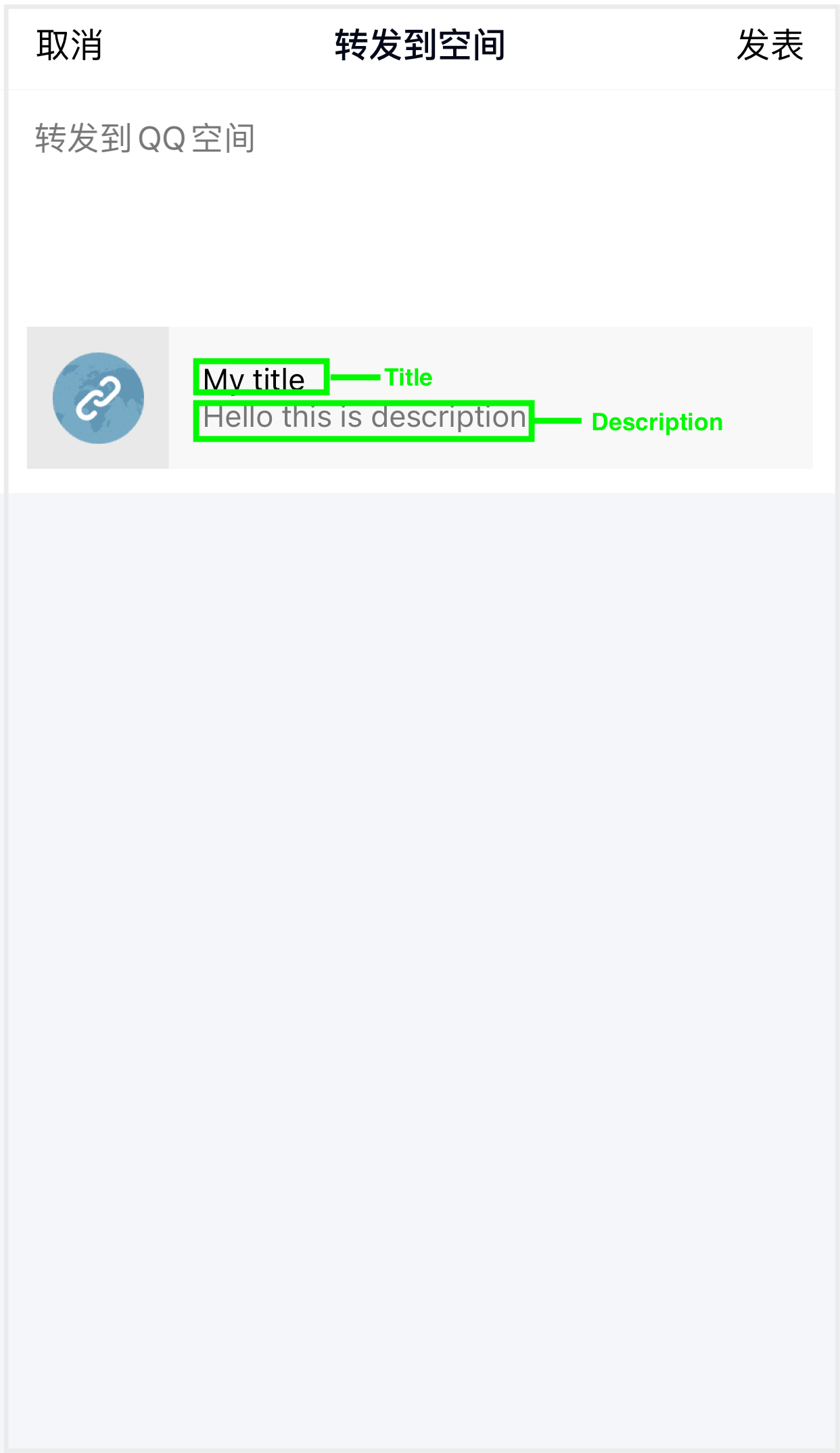
Share: Image
- Unity
- Unreal Engine
Call the Share
method, with Type
set to INTLFriendReqType.Friend_REQ_IMAGE
.
The player must have installed the QQ app on their device and is currently logged in.
Parameters:
Type
Description
: DescriptionImagePath
: Image file path, either the path of a local image file or an online URL
var reqInfo = new INTLFriendReqInfo ();
reqInfo.Type = (int)INTLFriendReqType.Friend_REQ_IMAGE;
reqInfo.Description = "INTL description";
reqInfo.ImagePath = "http://mat1.gtimg.com/www/qq2018/imgs/qq_logo_2018x2.png";
INTLAPI.Share(reqInfo, INTLChannel.QQ);
Call the Share
method, with Type
set to UINTLFriendReqType::kReqImage
.
The player must have installed the QQ app on their device and is currently logged in.
Parameters:
Type
Description
: DescriptionImagePath
: Image file path, either the path of a local image file or an online URL
FINTLFriendReqInfo reqInfo ;
reqInfo.Type = (int32)UINTLFriendReqType::kReqImage;
reqInfo.Description = "INTL description";
reqInfo.ImagePath = "http://mat1.gtimg.com/www/qq2018/imgs/qq_logo_2018x2.png";
UINTLSDKAPI::Share(reqInfo, EINTLLoginChannel::kChannelQQ);
Share: Video
- Unity
- Unreal Engine
Call the Share
method, with Type
set to INTLFriendReqType.Friend_REQ_VIDEO
.
The player must have installed the QQ app on their device and is currently logged in.
Parameters:
Type
Title
: TitleDescription
: DescriptionMediaPath
: Video file path, needs to be the URL of an online videoThumbPath
: Thumbnail file path
var reqInfo = new INTLFriendReqInfo ();
reqInfo.Type = (int)INTLFriendReqType.Friend_REQ_VIDEO;
reqInfo.Title = "Game Video";
reqInfo.Description = "Golden moment";
reqInfo.MediaPath = "http://v.qq.com/cover/5/53x6bbyb07ebl3s/n0013r8esy6.html";
reqInfo.ThumbPath = "http://mat1.gtimg.com/www/qq2018/imgs/qq_logo_2018x2.png";
INTLAPI.Share(reqInfo, INTLChannel.QQ);
Call the Share
method, with Type
set to UINTLFriendReqType::kReqVideo
.
The player must have installed the QQ app on their device and is currently logged in.
Parameters:
Type
Title
: TitleDescription
: DescriptionMediaPath
: Video file path, needs to be the URL of an online videoThumbPath
: Thumbnail file path
FINTLFriendReqInfo reqInfo ;
reqInfo.Type = (int32)UINTLFriendReqType::kReqVideo;
reqInfo.Title = "Game Video";
reqInfo.Description = "Golden moment";
reqInfo.MediaPath = "http://v.qq.com/cover/5/53x6bbyb07ebl3s/n0013r8esy6.html";
reqInfo.ThumbPath = "http://mat1.gtimg.com/www/qq2018/imgs/qq_logo_2018x2.png";
UINTLSDKAPI::Share(reqInfo, EINTLLoginChannel::kChannelQQ);
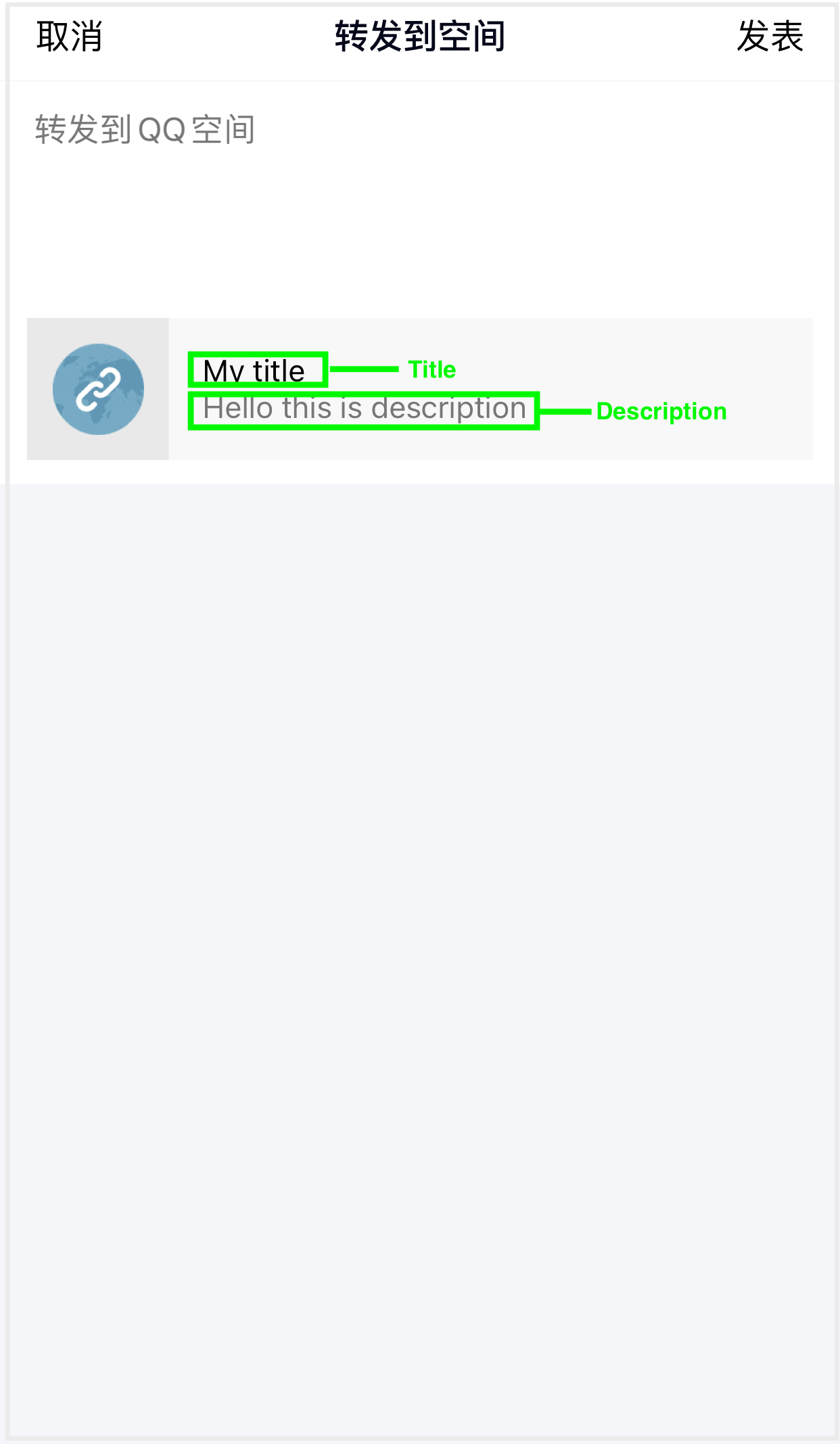
System
The native iOS system sharing supports sharing text messages, images, and links. System sharing also supports customizing the system share interface.
Share
The sharing features each have different required parameters, such as Description
for text sharing. If irrelevant parameters are entered, such as Link
or ImagePath
when sharing text, the irrelevant parameters are ignored.
The callback only indicates whether the system sharing interface was successfully opened and does not indicate whether the sharing was successful.
Feature | Required parameter |
---|---|
Share: Text | Description |
Share: Link | Description Link |
Share: Image | ImagePath |
Customize system share interface
When customizing the iOS system sharing interface, only iOS system apps, such as mail, reminders, SMS, AirDrop can be deleted. Games cannot select which apps are displayed. When customizing the iOS system interface, all social apps will be shown regardless of ExtraJson
configurations.
Report system sharing events
Player Network SDK will report the system sharing events for selected channels, and games can check the report insight_1000_system_share on DD Platform and filter keywords (such as QQ, Facebook, VK) when looking up the reports.
Key fields:
name
: Callback name, such asvk:com.vkontakte.android.sharing.SharingExternalActivity
package_name
: Channel package name, such ascom.tencent.mqq
for QQ
Twitter
As Twitter SDK is no longer being maintained, system sharing is used when sharing to Twitter.
The following content can be shared to Twitter using Share
:
- Share: Text
- Share: Link
- Share: Image
For a player to share game content to Twitter on iOS, they need to install and log in to the Twitter app on their device before sharing. However, Twitter occasionally fails to redirect to the game when players authorize the game in Twitter on iOS devices. Retrying can resolve the issue.
If a player has multiple Twitter accounts and has switched their login account before sharing game content, the previously logged-in account will be used for sharing. To resolve this issue, add the \"login_first\":1
property to ExtraJson
when calling Share
to make Twitter go through authorization each time before sharing (without changing the current login status of the game), ensuring that the content is shared with the current account.
Twitter may return a 403 error if the same content is shared repeatedly in a short time.
Share: Text
- Unity
- Unreal Engine
Call the Share
method, with Type
set to INTLFriendReqType.Friend_REQ_TEXT
.
The player must have installed the Twitter app on their device and is currently logged in.
Parameters:
Type
Description
: Text content
var reqInfo = new INTLFriendReqInfo
reqInfo.Type = (int)INTLFriendReqType.Friend_REQ_TEXT;
reqInfo.Description = "Hello, long time no see";
INTLAPI.Share(reqInfo, INTLChannel.Twitter);
Call the Share
method, with Type
set to UINTLFriendReqType::kReqText
.
The player must have installed the Twitter app on their device and is currently logged in.
Parameters:
Type
Description
: Text content
FINTLFriendReqInfo reqInfo;
reqInfo.Type = (int32)UINTLFriendReqType::kReqText;
reqInfo.Description = "Hello, long time no see";
UINTLSDKAPI::Share(reqInfo, EINTLLoginChannel::kChannelTwitter);
Share: Link
- Unity
- Unreal Engine
Call the Share
method, with Type
set to INTLFriendReqType.Friend_REQ_LINK
.
The player must have installed the Twitter app on their device and is currently logged in.
Parameters:
Type
Link
: URL
var reqInfo = new INTLFriendReqInfo
reqInfo.Type = (int)INTLFriendReqType.Friend_REQ_LINK;
reqInfo.Link = "https://www.google.com";
INTLAPI.Share(reqInfo, INTLChannel.Twitter);
Call the Share
method, with Type
set to UINTLFriendReqType::kReqLink
.
The player must have installed the Twitter app on their device and is currently logged in.
Parameters:
Type
Link
: URL
FINTLFriendReqInfo reqInfo;
reqInfo.Type = (int32)UINTLFriendReqType::kReqLink;
reqInfo.Link = "https://www.google.com";
UINTLSDKAPI::Share(reqInfo, EINTLLoginChannel::kChannelTwitter);
Share: Image
- Unity
- Unreal Engine
Call the Share
method, with Type
set to INTLFriendReqType.Friend_REQ_IMAGE
.
The player must have installed the Twitter app on their device and is currently logged in.
Parameters:
Type
ImagePath
: Image file path, either the path of a local image file or an online URL.
var reqInfo = new INTLFriendReqInfo
reqInfo.Type = (int)INTLFriendReqType.Friend_REQ_IMAGE;
reqInfo.ImagePath = "/path/to/image";
INTLAPI.Share(reqInfo, INTLChannel.Twitter);
Call the Share
method, with Type
set to UINTLFriendReqType::kReqImage
.
The player must have installed the Twitter app on their device and is currently logged in.
Parameters:
Type
ImagePath
: Image file path, either the path of a local image file or an online URL.
FINTLFriendReqInfo reqInfo;
reqInfo.Type = (int32)UINTLFriendReqType::kReqImage;
reqInfo.ImagePath = "/path/to/image";
UINTLSDKAPI::Share(reqInfo, EINTLLoginChannel::kChannelTwitter);
VK
The following content can be shared to VK using Share
:
- Share: Text
- Share: Link
- Share: Image
Share: Text
- Unity
- Unreal Engine
Call the Share
method, with Type
set to INTLFriendReqType.Friend_REQ_TEXT
.
Parameters:
Type
Description
: Text content
var reqInfo = new INTLFriendReqInfo
reqInfo.Type = (int)INTLFriendReqType.Friend_REQ_TEXT;
reqInfo.Description = "Hello, long time no see";
INTLAPI.Share(reqInfo, INTLChannel.VK);
Call the Share
method, with Type
set to UINTLFriendReqType::kReqText
.
Parameters:
Type
Description
: Text content
FINTLFriendReqInfo reqInfo;
reqInfo.Type = (int32)UINTLFriendReqType::kReqText;
reqInfo.Description = "Description";
UINTLSDKAPI::Share(reqInfo, EINTLLoginChannel::kChannelVK);
Share: Link
- Unity
- Unreal Engine
Call the Share
method, with Type
set to INTLFriendReqType.Friend_REQ_LINK
.
Parameters:
Type
Link
: URL
var reqInfo = new INTLFriendReqInfo
reqInfo.Type = (int)INTLFriendReqType.Friend_REQ_LINK;
reqInfo.Link = "https://www.google.com";
INTLAPI.Share(reqInfo, INTLChannel.VK);
Call the Share
method, with Type
set to UINTLFriendReqType::kReqLink
.
Parameters:
Type
Link
: URL
FINTLFriendReqInfo reqInfo;
reqInfo.Type = (int32)UINTLFriendReqType::kReqLink;
reqInfo.Link = "https://www.google.com";
UINTLSDKAPI::Share(reqInfo, EINTLLoginChannel::kChannelVK);
Share: Image
- Unity
- Unreal Engine
Call the Share
method, with Type
set to INTLFriendReqType.Friend_REQ_IMAGE
.
Parameters:
Type
ImagePath
: Image file path, either the path of a local image file or an online URL.
var reqInfo = new INTLFriendReqInfo
reqInfo.Type = (int)INTLFriendReqType.Friend_REQ_IMAGE;
reqInfo.ImagePath = "/path/to/image";
INTLAPI.Share(reqInfo, INTLChannel.VK);
Call the Share
method, with Type
set to UINTLFriendReqType::kReqImage
.
Parameters:
Type
ImagePath
: Image file path, either the path of a local image file or an online URL.
FINTLFriendReqInfo reqInfo;
reqInfo.Type = (int32)UINTLFriendReqType::kReqImage;
reqInfo.ImagePath = "/path/to/image";
UINTLSDKAPI::Share(reqInfo, EINTLLoginChannel::kChannelVK);
WhatsApp
The following content can be shared to WhatsApp using Share
:
- Share: Text
- Share: Link
- Share: Image
- Share: Video
When sharing text or links, Share
returns success immediately regardless of the actual result.
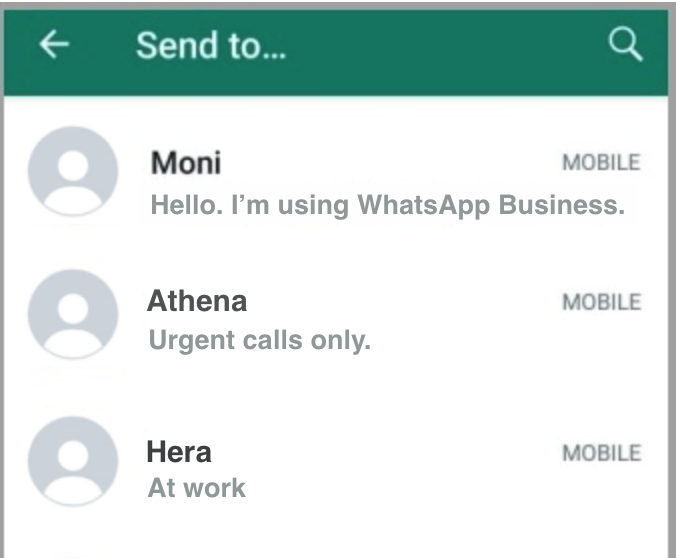
Share: Text
- Unity
- Unreal Engine
Call the Share
method, with Type
set to INTLFriendReqType.Friend_REQ_TEXT
.
The player must have installed the WhatsApp app on their device and is currently logged in.
Parameters:
Type
Description
: Text content
var reqInfo = new INTLFriendReqInfo
reqInfo.Type = (int)INTLFriendReqType.Friend_REQ_TEXT;
reqInfo.Description = "Hello, long time no see";
INTLAPI.Share(reqInfo, "WhatsApp");
Call the Share
method, with Type
set to UINTLFriendReqType::kReqText
.
The player must have installed the WhatsApp app on their device and is currently logged in.
Parameters:
Type
Description
: Text content
FINTLFriendReqInfo reqInfo;
reqInfo.Type = (int32)UINTLFriendReqType::kReqText;
reqInfo.Description = "Hello, long time no see";
UINTLSDKAPI::Share(reqInfo, "WhatsApp");
Share: Link
- Unity
- Unreal Engine
Call the Share
method, with Type
set to INTLFriendReqType.Friend_REQ_LINK
.
The player must have installed the WhatsApp app on their device and is currently logged in.
Parameters:
Type
Link
: URL
var reqInfo = new INTLFriendReqInfo
reqInfo.Type = (int)INTLFriendReqType.Friend_REQ_LINK;
reqInfo.Link = "https://www.google.com";
INTLAPI.Share(reqInfo, "WhatsApp");
Call the Share
method, with Type
set to UINTLFriendReqType::kReqLink
.
The player must have installed the WhatsApp app on their device and is currently logged in.
Parameters:
Type
Link
: URL
FINTLFriendReqInfo reqInfo;
reqInfo.Type = (int32)UINTLFriendReqType::kReqLink;
reqInfo.Link = "https://www.google.com";
UINTLSDKAPI::Share(reqInfo, "WhatsApp");
Share: Image
- Unity
- Unreal Engine
Call the Share
method, with Type
set to INTLFriendReqType.Friend_REQ_IMAGE
.
The player must have installed the WhatsApp app on their device and is currently logged in.
Parameters:
Type
ImagePath
: Image file path
var reqInfo = new INTLFriendReqInfo
reqInfo.Type = (int)INTLFriendReqType.Friend_REQ_IMAGE;
reqInfo.ImagePath = "/path/to/image";
INTLAPI.Share(reqInfo, "WhatsApp");
Call the Share
method, with Type
set to UINTLFriendReqType::kReqImage
.
The player must have installed the WhatsApp app on their device and is currently logged in.
Parameters:
Type
ImagePath
: Image file path
FINTLFriendReqInfo reqInfo;
reqInfo.Type = (int32)UINTLFriendReqType::kReqImage;
reqInfo.ImagePath = "/path/to/image";
UINTLSDKAPI::Share(reqInfo, "WhatsApp");
Share: Video
- Unity
- Unreal Engine
Call the Share
method, with Type
set to INTLFriendReqType.Friend_REQ_VIDEO
.
The player must have installed the WhatsApp app on their device and is currently logged in.
Parameters:
Type
MediaPath
: Video file path, either the path of a local video file or an online URL.
var reqInfo = new INTLFriendReqInfo
reqInfo.Type = (int)INTLFriendReqType.Friend_REQ_VIDEO;
reqInfo.MediaPath = "/path/to/video";
INTLAPI.Share(reqInfo, "WhatsApp");
Call the Share
method, with Type
set to UINTLFriendReqType::kReqVideo
.
The player must have installed the WhatsApp app on their device and is currently logged in.
Parameters:
Type
MediaPath
: Video file path, either the path of a local video file or an online URL.
FINTLFriendReqInfo reqInfo;
reqInfo.Type = (int32)UINTLFriendReqType::kReqVideo;
reqInfo.MediaPath = "/path/to/video";
UINTLSDKAPI::Share(reqInfo, "WhatsApp");
Youtube
The following content can be shared to Youtube using Share
:
- Share: Video
Share: Video
- Unity
- Unreal Engine
Call the Share
method, with Type
set to INTLFriendReqType.Friend_REQ_VIDEO
.
Parameters:
Type
MediaPath
: Video file path, either the path of a local video file or an online URL.
var reqInfo = new INTLFriendReqInfo
reqInfo.Type = (int)INTLFriendReqType.Friend_REQ_VIDEO;
reqInfo.MediaPath = "/path/to/video";
INTLAPI.Share(reqInfo, "Youtube");
Call the Share
method, with Type
set to UINTLFriendReqType::kReqVideo
.
Parameters:
Type
MediaPath
: Video file path, either the path of a local video file or an online URL.
FINTLFriendReqInfo reqInfo;
reqInfo.Type = (int32)UINTLFriendReqType::kReqVideo;
reqInfo.MediaPath = "/path/to/video";
UINTLSDKAPI::Share(reqInfo, "Youtube");
TikToK
The following content can be shared to TikToK using Share
:
- Share: Video
Share: Video
- Unity
- Unreal Engine
Call the Share
method, with Type
set to INTLFriendReqType.Friend_REQ_VIDEO
.
The player must have installed the TikToK app on their device and is currently logged in.
Parameters:
Type
MediaPath
: Video file path, either the path of a local video file or an online URL.
var reqInfo = new INTLFriendReqInfo
reqInfo.Type = (int)INTLFriendReqType.Friend_REQ_VIDEO;
reqInfo.MediaPath = "/path/to/video";
INTLAPI.Share(reqInfo, "TikToK");
Call the Share
method, with Type
set to UINTLFriendReqType::kReqVideo
.
The player must have installed the TikToK app on their device and is currently logged in.
Parameters:
Type
MediaPath
: Video file path, either the path of a local video file or an online URL.
FINTLFriendReqInfo reqInfo;
reqInfo.Type = (int32)UINTLFriendReqType::kReqVideo;
reqInfo.MediaPath = "/path/to/video";
UINTLSDKAPI::Share(reqInfo, "TikToK");
Callbacks
- Unity
- Unreal Engine
API | Function |
---|---|
AddFriendBaseResultObserver | Adds the BaseResult callback to manage SendMessage and Share callbacks. |
RemoveFriendBaseResultObserver | Delete the BaseResult callback. |
API | Function |
---|---|
SetFriendBaseResultObserver | Sets BaseResult callback for SendMessage and Share. |
GetFriendBaseResultObserver | Gets BaseResult callback for SendMessage and Share. |
OnFriendBaseResult_Implementation | Implements BaseResult callbacks for SendMessage and Share. |