QR Code Plugin
The QR code plugin provides useful functions for QR code operations in your app, including methods to open the QR code scanner, display a QR code, and customize an icon and position marker color for the QR code.
The QR code scanner interface consists of 3 main features:
- My QR code: To generate and display a QR code.
- Select from album: To select a QR code image from the system album.
- Turn on torch light: To toggle the device flashlight, automatically displayed when the surrounding brightness is low.
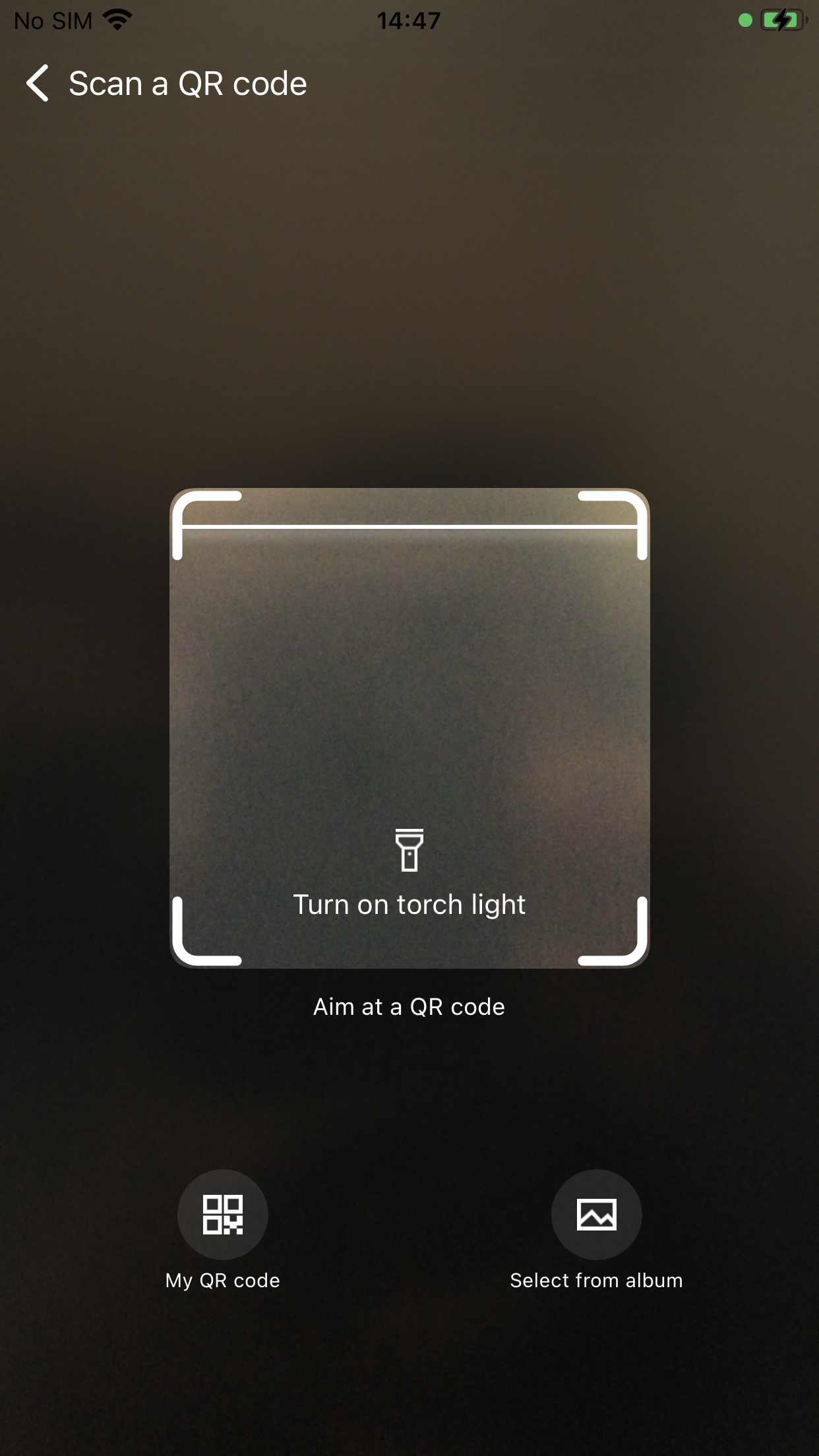
For iOS 14.0+ and Android 14+, when app access to the system album is restricted, only photos selected by the player will be shown when Select from album is clicked.
Album with limited photo access:
Prerequisites
2. iOS configurations
For iOS on Unity, set up Game Center as an identity provider first before implementing the QR code plugin.
Add the following keys and values to your project's Info.plist
file:
UISupportsRightToLeft
: Enables right-to-left language support.CFBundleAllowMixedLocalizations
: Allows mixed localizations.PHPhotoLibraryPreventAutomaticLimitedAccessAlert
: Prevents the limited photos access alert.NSPhotoLibraryUsageDescription
: Describes why the app needs access to the photo library.NSCameraUsageDescription
: Describes why the app needs access to the camera.<plist version="1.0">
<dict>
<key>UISupportsRightToLeft</key>
<true/>
<key>CFBundleAllowMixedLocalizations</key>
<true/>
<key>PHPhotoLibraryPreventAutomaticLimitedAccessAlert</key>
<true/>
<key>NSPhotoLibraryUsageDescription</key>
<string>This app requires access to your photo library to upload and share your photos.</string>
<key>NSCameraUsageDescription</key>
<string>This app requires access to your camera to capture photos and videos for your profile and posts.</string>
</dict>
</plist>
Register Callbacks
Add the following observers to handle callbacks:
- Unity
- Unreal Engine
// Add callbacks
public void AddQRCodeObserver()
{
INTLAPI.AddQRCodeResultObserver(OnQRCodeResultEvent);
}
// Remove callbacks
public void RemoveQRCodeObserver()
{
INTLAPI.RemoveQRCodeResultObserver(OnQRCodeResultEvent);
}
// Process the INTLQRCodeResult callback
private void OnQRCodeResultEvent(INTLQRCodeResult qrCodeRet)
{
Debug.Log("OnQRCodeResultEvent");
string methodTag = "";
if (qrCodeRet.MethodId == (int)INTLMethodID.INTL_QRCODE_OPEN_SCANNER)
{
methodTag = "OpenQRScanner";
}
else if (qrCodeRet.MethodId == (int)INTLMethodID.INTL_QRCODE_SHOW)
{
methodTag = "ShowQRCode";
}
else if (qrCodeRet.MethodId == (int)INTLMethodID.INTL_QRCODE_SET_ICON)
{
methodTag = "SetIcon";
}
else if (qrCodeRet.MethodId == (int)INTLMethodID.INTL_QRCODE_SET_POSITION_MARKER_COLOR)
{
methodTag = "SetPositionMarkerColor";
}
}
// Add callbacks
FINTLQRCodeEvent QRCodeEvent;
QRCodeEvent.AddUObject(this, &UQRCodeWindow::OnQRCodeResult_Implementation);
UINTLSDKAPI::SetQRCodeResultObserver(QRCodeEvent);
// Remove callbacks
UINTLSDKAPI::GetQRCodeResultObserver().Clear();
// Process the FINTLQRCodeResult callback
void UQRCodeWindow::OnQRCodeResult_Implementation(FINTLQRCodeResult qrCodeRet)
{
UE_LOG(LogTemp, Log, TEXT("==== OnQRCodeResultEvent"));
FString methodTag;
if (qrCodeRet.MethodId == INTL_QRCODE_OPEN_SCANNER)
{
methodTag = TEXT("OpenQRScanner");
}
else if (qrCodeRet.MethodId == INTL_QRCODE_SHOW)
{
methodTag = TEXT("ShowQRCode");
}
else if (qrCodeRet.MethodId == INTL_QRCODE_SET_ICON)
{
methodTag = TEXT("SetIcon");
}
else if (qrCodeRet.MethodId == INTL_QRCODE_SET_POSITION_MARKER_COLOR)
{
methodTag = TEXT("SetPositionMarkerColor");
}
}
OpenQRScanner
Opens the QR code scanner with the specified parameters.
qrCodeContent
: JSON string for QR code generation, to be customized according to your requirements.
- Unity
- Unreal Engine
string qrCodeContent = "{\
\"deeplink\": \"https://example.com/path\", \
\"openid\": \"user-12345\", \
\"additionalKey1\": \"value1\"\
}";
INTLAPI.OpenQRScanner(qrCodeContent);
FString qrCodeContent = TEXT("{\"deeplink\":\"https://example.com/path\", "
"\"openid\":\"user-12345\", "
"\"additionalKey1\":\"value1\"}");
UINTLSDKAPI::OpenQRScanner(qrCodeContent);
ShowQRCode
Displays the specified QR code content. The default style for the QR code may vary slightly based on the platform.
qrCodeContent
: JSON string for QR code generation, to be customized according to your requirements.
- Unity
- Unreal Engine
string qrCodeContent = "{\
\"deeplink\": \"https://example.com/path\", \
\"openid\": \"user-12345\", \
\"additionalKey1\": \"value1\"\
}";
INTLAPI.ShowQRCode(qrCodeContent);
FString qrCodeContent = TEXT("{\"deeplink\":\"https://example.com/path\", "
"\"openid\":\"user-12345\", "
"\"additionalKey1\":\"value1\"}");
UINTLSDKAPI::ShowQRCode(qrCodeContent);
Android default QR code display:
iOS default QR code display:
SetIcon
Sets an icon for the generated QR code.
imageURL
: URL of the icon.
- Unity
- Unreal Engine
string imageURL = "https://example.com/icon.png";
INTLAPI.SetIcon(imageURL);
FString imageURL = TEXT("https://example.com/icon.png");
UINTLSDKAPI::SetIcon(imageURL);
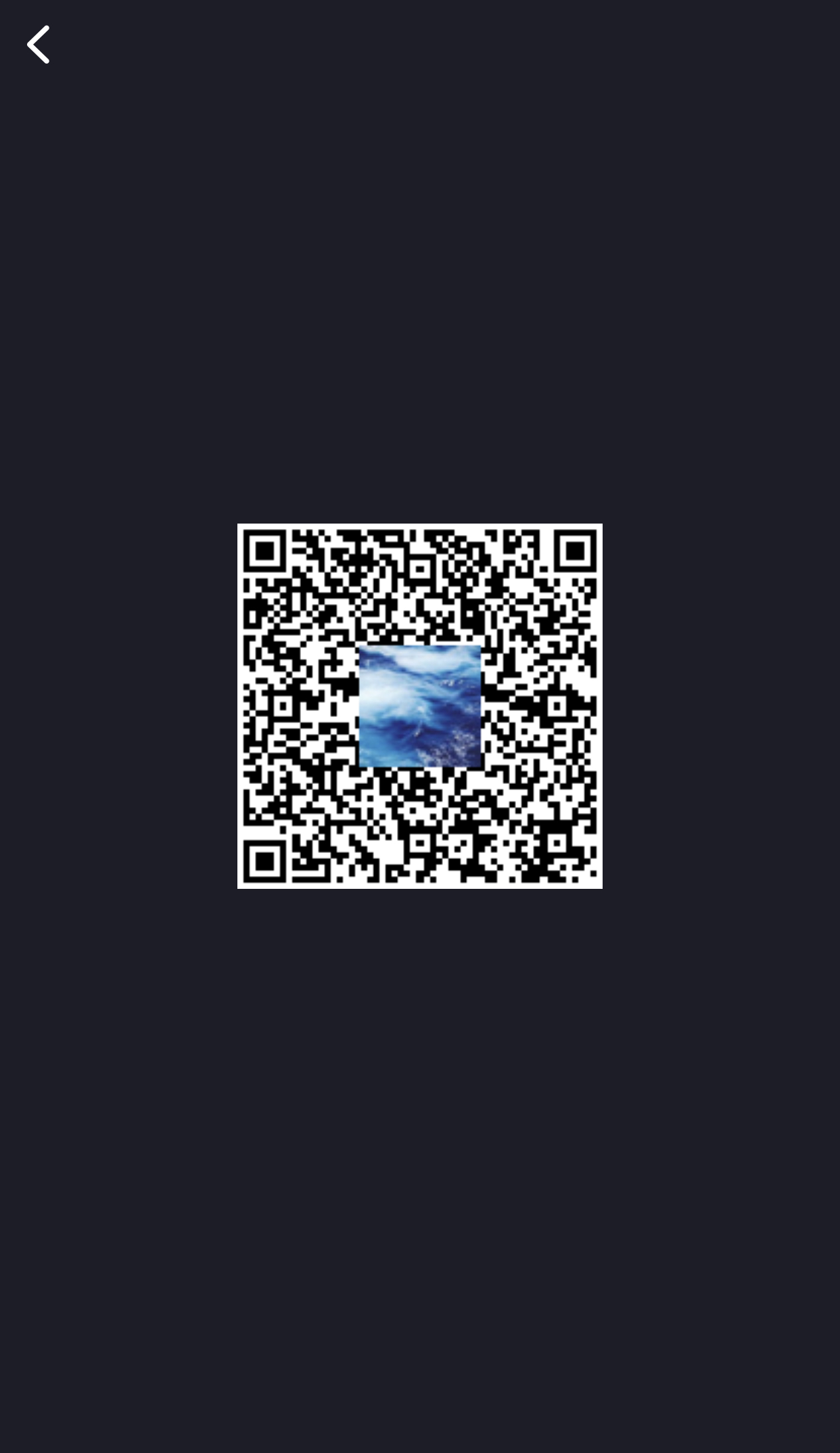
SetPositionMarkerColor
Sets the position marker color for the QR code.
hexCode
: Hexadecimal string representing the color of the position marker, such as#FF5733
.
- Unity
- Unreal Engine
string hexCode = "#FF5733";
INTLAPI.SetPositionMarkerColor(hexCode);
FString hexCode = TEXT("#FF5733");
UINTLSDKAPI::SetPositionMarkerColor(hexCode);
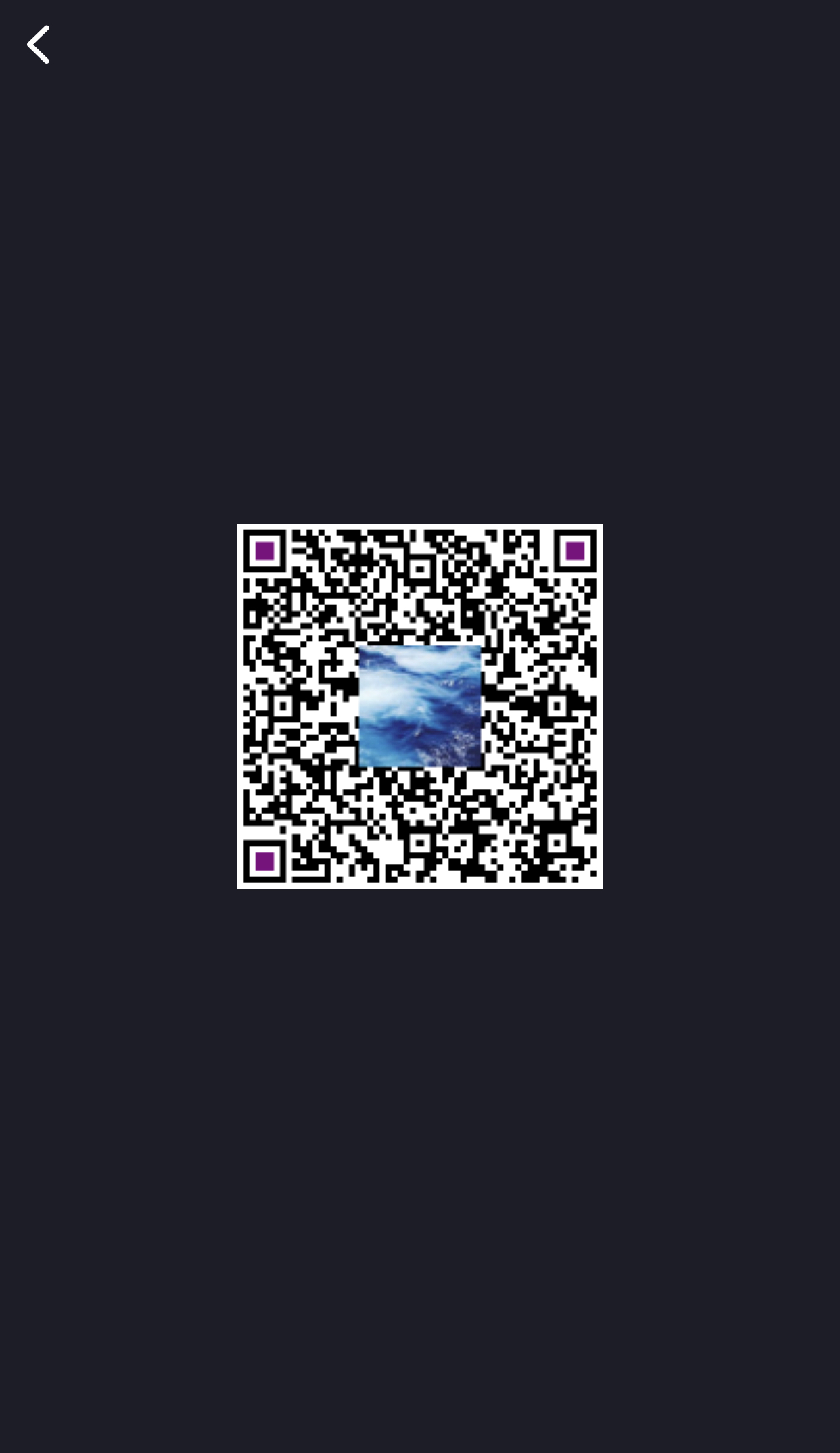